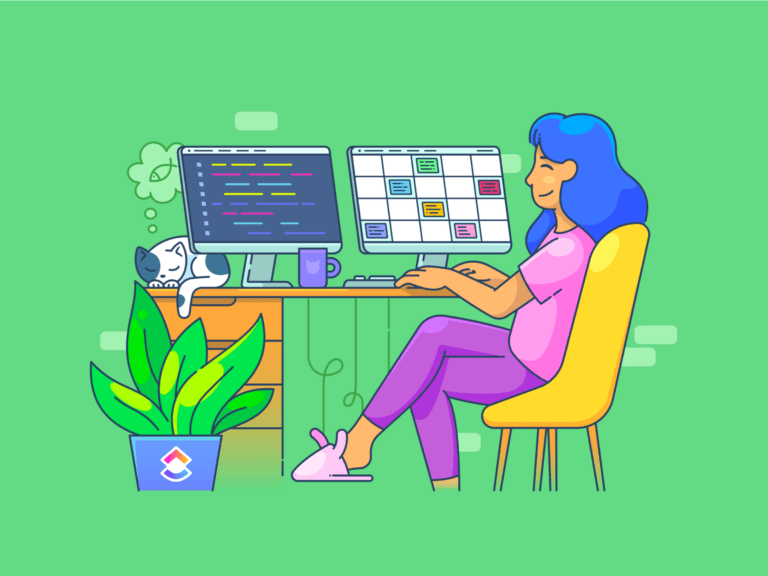
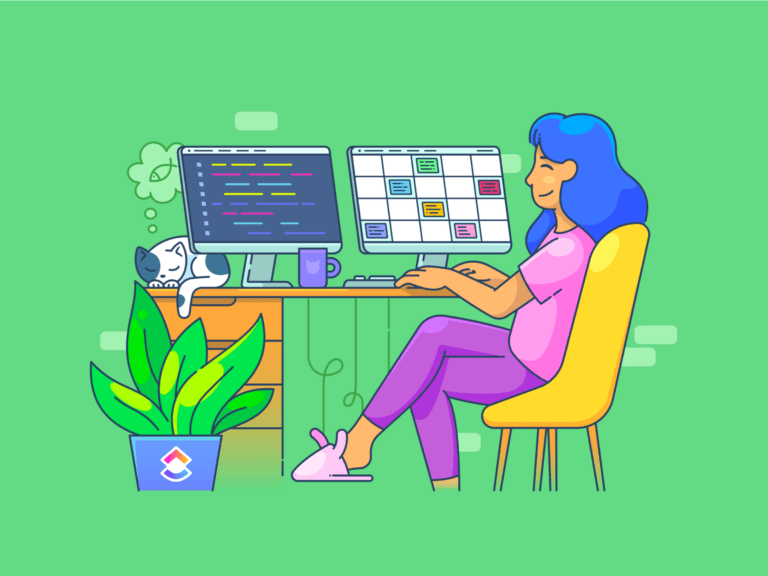
Virtual workspaces like Slack have become a big part of our work culture.
Need to communicate with your teammates? Just send them a direct message on Slack. Want to collaborate on a project? Slack has you covered with channels, integrations, and easy file sharing.
One of Slack’s best capabilities is its automated bots, which perform three major roles when integrated into a Slack group or channel—automating tasks, sending notifications, and answering questions.
Sounds like a lifesaver, right? In this article, we’ll explore how to create the perfect Slack bot that suits your team’s needs.
❗Note: Before you begin, you’ll need the Slack CLI installed and your new workspace authorized. Run Slack auth list to verify this.
- How to Make Your Own Slack Chatbot
- Step 1: Create a Slack app using the CLI
- Step 2: Create the app mandate
- Step 3: Create a workflow for setting up the welcome message
- Step 4: Use a datastore to store the welcome message
- Step 5: Create a custom function to store the welcome message in the datastore
- Step 6: Create triggers to activate workflows
- Step 7: Create a workflow for sending the welcome message
- Step 8: Create a custom function to send the welcome message
- Step 9: Use your Slack app
- Step 10: Your Slack bot is ready!
- Limitations of Using Slack Bots for Communication
- Enhancing Team Communication with ClickUp and Slack
- ClickUp Does What Slack Can, and More
How to Make Your Own Slack Chatbot
Let’s walk through the steps needed to create and integrate a Slack bot.
For your ease, we’ll lead with an example—how to write a Slack bot that sends welcome text to new users. Do note that the process is more or less similar to other bot functions.
Each step requires some coding. But don’t worry—this guide will walk you through every detail to ensure you can create your chatbot without any hassle.
Step 1: Create a Slack app using the CLI
Before you dive into building your Slack bot, you’ll need the right tools in place. The first step involves creating your Slack app using the command-line interface (CLI).
Begin by setting up a blank app with the Slack CLI using this command:
slack create welcome-bot-app –template https://github.com/slack-samples/deno-welcome-bot
Running this command will create an app folder linked to your Slack account. Inside your new app folder, create three key directories that will serve as the foundation for your Slack bot:
- functions
- workflows
- triggers
With these folders and permissions in place, you’re ready to start building your Slack app!
Alternatively, instead of writing all the code yourself, you can use the following command to create the Welcome Bot app directly:
slack create welcome-bot-app --template https://github.com/slack-samples/deno-welcome-bot
Once your project is created, navigate to your project directory to customize your Slack bot.
Step 2: Create the app mandate
The app manifest is your blueprint for the Slack bot. For a Welcome Bot, your manifest will include:
Workflows:
- MessageSetupWorkflow
- SendWelcomeMessageWorkflow
Datastore:
- WelcomeMessageDatastore
Scopes:
- chat:write
- chat:write.public
- datastore:read
- datastore:write
- channels:read
- triggers:write
- triggers:read
When you bring all these components together, your manifest.ts file will resemble something like this:
// /manifest.ts import { Manifest } from "deno-slack-sdk/mod.ts"; import { WelcomeMessageDatastore } from "./datastores/messages.ts"; import { MessageSetupWorkflow } from "./workflows/create_welcome_message.ts"; import { SendWelcomeMessageWorkflow } from "./workflows/send_welcome_message.ts"; export default Manifest({ name: "Welcome Message Bot", description: "Quick way to setup automated welcome messages for channels in your workspace.", icon: "assets/default_new_app_icon.png", workflows: [MessageSetupWorkflow, SendWelcomeMessageWorkflow], outgoingDomains: [], datastores: [WelcomeMessageDatastore], botScopes: [ "chat:write", "chat:write.public", "datastore:read", "datastore:write", "channels:read", "triggers:write", "triggers:read", ], });
Step 3: Create a workflow for setting up the welcome message
First, we start by defining the workflow:
- Create file: Add a new file named create_welcome_message.ts in the workflows folder
- Define workflow: In this file, define the MessageSetupWorkflow to allow a bot user to set up a welcome message via a form
Here’s how the workflow definition will look:
// /workflows/create_welcome_message.ts import { DefineWorkflow, Schema } from "deno-slack-sdk/mod.ts"; import { WelcomeMessageSetupFunction } from "../functions/create_welcome_message.ts"; /** * The MessageSetupWorkflow opens a form where the user creates a * welcome message. The trigger for this workflow is found in * `/triggers/welcome_message_trigger.ts` */ export const MessageSetupWorkflow = DefineWorkflow({ callback_id: "message_setup_workflow", title: "Create Welcome Message", description: " Creates a message to welcome new users into the channel.", input_parameters: { properties: { interactivity: { type: Schema.slack.types.interactivity, }, channel: { type: Schema.slack.types.channel_id, }, }, required: ["interactivity"], }, });
Next, we add a form:
- Use OpenForm: Add a form using the OpenForm function to collect bot user inputs
Here’s how to add the OpenForm function to your create_welcome_message.ts workflow:
// /workflows/create_welcome_message.ts /** * This step uses the OpenForm Slack function. The form has two * inputs -- a welcome message and a channel id for that message to * be posted in. */ const SetupWorkflowForm = MessageSetupWorkflow.addStep( Schema.slack.functions.OpenForm, { title: "Welcome Message Form", submit_label: "Submit", description: ":wave: Create a welcome message for a channel!", interactivity: MessageSetupWorkflow.inputs.interactivity, fields: { required: ["channel", "messageInput"], elements: [ { name: "messageInput", title: "Your welcome message", type: Schema.types.string, long: true, }, { name: "channel", title: "Select a channel to post this message in", type: Schema.slack.types.channel_id, default: MessageSetupWorkflow.inputs.channel, }, ], }, }, );
Then, we add confirmation.
Add the following step to your create_welcome_message.ts workflow:
// /workflows/create_welcome_message.ts /** * This step takes the form output and passes it along to a custom * function which sets the welcome message up. * See `/functions/setup_function.ts` for more information. */ MessageSetupWorkflow.addStep(WelcomeMessageSetupFunction, { message: SetupWorkflowForm.outputs.fields.messageInput, channel: SetupWorkflowForm.outputs.fields.channel, author: MessageSetupWorkflow.inputs.interactivity.interactor.id, }); /** * This step uses the SendEphemeralMessage Slack function. * An ephemeral confirmation message will be sent to the user * creating the welcome message, after the user submits the above * form. */ MessageSetupWorkflow.addStep(Schema.slack.functions.SendEphemeralMessage, { channel_id: SetupWorkflowForm.outputs.fields.channel, user_id: MessageSetupWorkflow.inputs.interactivity.interactor.id, message: `Your welcome message for this channel was successfully created! :white_check_mark:`, }); export default MessageSetupWorkflow;
Step 4: Use a datastore to store the welcome message
Start with creating a datastore.
- Create file: In your datastores folder, create a file named messages.ts
- Define structure: Set up the datastore structure to store welcome messages
In this file, you’ll define the structure of the datastore where the text field welcome messages will be stored:
// /datastores/messages.ts import { DefineDatastore, Schema } from "deno-slack-sdk/mod.ts"; /** * Datastores are a Slack-hosted location to store * and retrieve data for your app. * https://api.slack.com/automation/datastores */ export const WelcomeMessageDatastore = DefineDatastore({ name: "messages", primary_key: "id", attributes: { id: { type: Schema.types.string, }, channel: { type: Schema.slack.types.channel_id, }, message: { type: Schema.types.string, }, author: { type: Schema.slack.types.user_id, }, }, });
Step 5: Create a custom function to store the welcome message in the datastore
First, define the custom function.
- Create file: In the functions folder, create a file named create_welcome_message.ts
- Define function: Add the following code to define the function for storing the welcome message
Here’s the code to define the custom function:
// /functions/create_welcome_message.ts import { DefineFunction, Schema, SlackFunction } from "deno-slack-sdk/mod.ts"; import { SlackAPIClient } from "deno-slack-sdk/types.ts"; import { SendWelcomeMessageWorkflow } from "../workflows/send_welcome_message.ts"; import { WelcomeMessageDatastore } from "../datastores/messages.ts"; /** * This custom function will take the initial form input, store it * in the datastore and create an event trigger to listen for * user_joined_channel events in the specified channel. */ export const WelcomeMessageSetupFunction = DefineFunction({ callback_id: "welcome_message_setup_function", title: "Welcome Message Setup", description: "Takes a welcome message and stores it in the datastore", source_file: "functions/create_welcome_message.ts", input_parameters: { properties: { message: { type: Schema.types.string, description: "The welcome message", }, channel: { type: Schema.slack.types.channel_id, description: "Channel to post in", }, author: { type: Schema.slack.types.user_id, description: "The user ID of the person who created the welcome message", }, }, required: ["message", "channel"], }, });
Next, add your required functionality.
- Store data: Include a code to save the welcome message details in your datastore and set up bot events triggers
// /functions/create_welcome_message.ts export default SlackFunction( WelcomeMessageSetupFunction, async ({ inputs, client }) => { const { channel, message, author } = inputs; const uuid = crypto.randomUUID(); // Save information about the welcome message to the datastore const putResponse = await client.apps.datastore.put< typeof WelcomeMessageDatastore.definition >({ datastore: WelcomeMessageDatastore.name, item: { id: uuid, channel, message, author }, }); if (!putResponse.ok) { return { error: `Failed to save welcome message: ${putResponse.error}` }; } // Search for any existing triggers for the welcome workflow const triggers = await findUserJoinedChannelTrigger(client, channel); if (triggers.error) { return { error: `Failed to lookup existing triggers: ${triggers.error}` }; } // Create a new user_joined_channel trigger if none exist if (!triggers.exists) { const newTrigger = await saveUserJoinedChannelTrigger(client, channel); if (!newTrigger.ok) { return { error: `Failed to create welcome trigger: ${newTrigger.error}`, }; } } return { outputs: {} }; }, );
Then, integrate the Slack bot into your workflow.
- Update workflow: In your create_welcome_message.ts file, add a step to call the custom function
// /workflows/create_welcome_message.ts /** * This step takes the form output and passes it along to a custom * function which sets the welcome message up. * See `/functions/setup_function.ts` for more information. */ MessageSetupWorkflow.addStep(WelcomeMessageSetupFunction, { message: SetupWorkflowForm.outputs.fields.messageInput, channel: SetupWorkflowForm.outputs.fields.channel, author: MessageSetupWorkflow.inputs.interactivity.interactor.id, }); export default MessageSetupWorkflow;
With this step, your workflow is now capable of:
- Allowing a bot user to input and submit a welcome message through a form
- Storing the welcome message information in a data store
- Setting up triggers to ensure the welcome message is delivered when a new user joins the specified channel
Step 6: Create triggers to activate workflows
First, create a link trigger.
- Create file: In the triggers folder, create create_welcome_message_shortcut.ts
- Add code: Define the link trigger to start the MessageSetupWorkflow when a bot user clicks a specific link
Your code will look something like this.
// triggers/create_welcome_message_shortcut.ts import { Trigger } from "deno-slack-api/types.ts"; import MessageSetupWorkflow from "../workflows/create_welcome_message.ts"; import { TriggerContextData, TriggerTypes } from "deno-slack-api/mod.ts"; /** * This link trigger prompts the MessageSetupWorkflow workflow. */ const welcomeMessageTrigger: Trigger<typeof MessageSetupWorkflow.definition> = { type: TriggerTypes.Shortcut, name: "Setup a Welcome Message", description: "Creates an automated welcome message for a given channel.", workflow: `#/workflows/${MessageSetupWorkflow.definition.callback_id}`, inputs: { interactivity: { value: TriggerContextData.Shortcut.interactivity, }, channel: { value: TriggerContextData.Shortcut.channel_id, }, }, }; export default welcomeMessageTrigger;
Now, create the event trigger. This is essentially where you enable events that activate the bot. In this example, the bot user event trigger will be a new user joining a channel.
- Update file: Add the event trigger to create_welcome_message.ts to send the welcome message when a new user joins a channel
Here’s the code to add.
// /functions/create_welcome_message.ts /** * findUserJoinedChannelTrigger returns if the user_joined_channel trigger * exists for the "Send Welcome Message" workflow in a channel. */ export async function findUserJoinedChannelTrigger( client: SlackAPIClient, channel: string, ): Promise<{ error?: string; exists?: boolean }> { // Collect all existing triggers created by the app const allTriggers = await client.workflows.triggers.list({ is_owner: true }); if (!allTriggers.ok) { return { error: allTriggers.error }; } // Find user_joined_channel triggers for the "Send Welcome Message" // workflow in the specified channel const joinedTriggers = allTriggers.triggers.filter((trigger) => ( trigger.workflow.callback_id === SendWelcomeMessageWorkflow.definition.callback_id && trigger.event_type === "slack#/events/user_joined_channel" && trigger.channel_ids.includes(channel) )); // Return if any matching triggers were found const exists = joinedTriggers.length > 0; return { exists }; } /** * saveUserJoinedChannelTrigger creates a new user_joined_channel trigger * for the "Send Welcome Message" workflow in a channel. */ export async function saveUserJoinedChannelTrigger( client: SlackAPIClient, channel: string, ): Promise<{ ok: boolean; error?: string }> { const triggerResponse = await client.workflows.triggers.create< typeof SendWelcomeMessageWorkflow.definition >({ type: "event", name: "User joined channel", description: "Send a message when a user joins the channel", workflow: `#/workflows/${SendWelcomeMessageWorkflow.definition.callback_id}`, event: { event_type: "slack#/events/user_joined_channel", channel_ids: [channel], }, inputs: { channel: { value: channel }, triggered_user: { value: "{{data.user_id}}" }, }, }); if (!triggerResponse.ok) { return { ok: false, error: triggerResponse.error }; } return { ok: true }; }
Result:
- Link trigger: Allows bot users to set up a welcome message by clicking a link, initiating the MessageSetupWorkflow
- Event trigger: Sends the automated messages when a new user joins the specified channel, using the stored message and channel details
Step 7: Create a workflow for sending the welcome message
- Navigate to folder: Go to the workflows folder
- Create file: Create a new file named send_welcome_message.ts
- Add code: Define the workflow to retrieve and send the welcome message from the datastore
// /workflows/send_welcome_message.ts import { DefineWorkflow, Schema } from "deno-slack-sdk/mod.ts"; import { SendWelcomeMessageFunction } from "../functions/send_welcome_message.ts"; /** * The SendWelcomeMessageWorkFlow will retrieve the welcome message * from the datastore and send it to the specified channel, when * a new user joins the channel. */ export const SendWelcomeMessageWorkflow = DefineWorkflow({ callback_id: "send_welcome_message", title: "Send Welcome Message", description: "Posts an ephemeral welcome message when a new user joins a channel.", input_parameters: { properties: { channel: { type: Schema.slack.types.channel_id, }, triggered_user: { type: Schema.slack.types.user_id, }, }, required: ["channel", "triggered_user"], }, });
This workflow retrieves the stored welcome message and sends it to the appropriate channel when a new user joins, enhancing engagement and automating the onboarding process.
Step 8: Create a custom function to send the welcome message
1. Define the custom function:
- Navigate to folder: Go to the functions folder
- Create file: Create a new file named send_welcome_message.ts
2. Add function definition:
// /functions/send_welcome_message.ts import { DefineFunction, Schema, SlackFunction } from "deno-slack-sdk/mod.ts"; import { WelcomeMessageDatastore } from "../datastores/messages.ts"; /** * This custom function will pull the stored message from the datastore * and send it to the joining user as an ephemeral message in the * specified channel. */ export const SendWelcomeMessageFunction = DefineFunction({ callback_id: "send_welcome_message_function", title: "Sending the Welcome Message", description: "Pull the welcome messages and sends it to the new user", source_file: "functions/send_welcome_message.ts", input_parameters: { properties: { channel: { type: Schema.slack.types.channel_id, description: "Channel where the event was triggered", }, triggered_user: { type: Schema.slack.types.user_id, description: "User that triggered the event", }, }, required: ["channel", "triggered_user"], }, });
3. Add functionality:
- Implement Code: Add the following code below the function definition in send_welcome_message.ts:
// /functions/send_welcome_message.ts export default SlackFunction(SendWelcomeMessageFunction, async ( { inputs, client }, ) => { // Querying datastore for stored messages const messages = await client.apps.datastore.query< typeof WelcomeMessageDatastore.definition >({ datastore: WelcomeMessageDatastore.name, expression: "#channel = :mychannel", expression_attributes: { "#channel": "channel" }, expression_values: { ":mychannel": inputs.channel }, }); if (!messages.ok) { return { error: `Failed to gather welcome messages: ${messages.error}` }; } // Send the stored messages ephemerally for (const item of messages["items"]) { const message = await client.chat.postEphemeral({ channel: item["channel"], text: item["message"], user: inputs.triggered_user, }); if (!message.ok) { return { error: `Failed to send welcome message: ${message.error}` }; } } return { outputs: {}, }; });
4. Integrate custom function:
- Add to the workflow: Go back to your send_welcome_message.ts workflow file and add the custom function as a step:
// /workflows/send_welcome_message.ts SendWelcomeMessageWorkflow.addStep(SendWelcomeMessageFunction, { channel: SendWelcomeMessageWorkflow.inputs.channel, triggered_user: SendWelcomeMessageWorkflow.inputs.triggered_user, });
With this custom function, your Slack app settings will now send messages to any user who joins a private or public channel with a specific channel.
Step 9: Use your Slack app
1. Run your Slack app locally
- Install locally:
- Open your terminal and navigate to your app’s root folder
- Run: slack run
- Follow the terminal prompts to set up your local server
- Invoke the Link Trigger:
- Open a new terminal tab
- Create the link trigger: slack trigger create –trigger-def triggers/create_welcome_message_shortcut.ts
- Install the trigger in your workspace and choose the Local environment
- Copy the Shortcut URL provided
- Post this URL in a Slack channel to start the workflow and create the welcome message
📚 App Manifest Created app manifest for "welcomebot (local)" in "myworkspace" workspace ⚠️ Outgoing domains No allowed outgoing domains are configured If your function makes network requests, you will need to allow the outgoing domains Learn more about upcoming changes to outgoing domains: https://api.slack.com/changelog 🏠 Workspace Install Installed "welcomebot (local)" app to "myworkspace" workspace Finished in 1.5s ⚡ Trigger created Trigger ID: Ft0123ABC456 Trigger Type: shortcut Trigger Name: Setup a Welcome Message Shortcut URL: https://slack.com/shortcuts/Ft0123ABC456/XYZ123 ...
2. Launch your Slack app
- Deploy Slack:
- Run: slack deploy
- Recreate the Trigger:
- Create the trigger for the deployed app: slack trigger create –trigger-def triggers/create_welcome_message_shortcut.ts
- Choose the deployed option
- Use in a Deployed Environment:
- Copy the new Shortcut URL
- Use it within your Slack workspace
Step 10: Your Slack bot is ready!
Congratulations on reaching the final step of building your Slack bot! Your bot will now automatically send messages to new users. Test it out to make sure it’s working properly on a public channel.
Limitations of Using Slack Bots for Communication
By now, you must have realized the convenience Slack bots bring to workplace communication. After all, who doesn’t love a little help with automation?
But there are a few limitations you should be wary of.
Knowing these limitations will allow you to make an informed decision about which workplace communication platform is most efficient for you.
1. Slack bots can be distracting
Slack bots can be a double-edged sword when it comes to focus.
Sure, it provides quick information and helps with tasks with a simple query. But it also comes with a barrage of notifications, from the bot’s token updates to team messages.
This constant distraction can hinder work. It often makes it difficult for your employees to prioritize work with the additional bot noise.
2. Slack can be expensive, and so can its bots
This is probably the biggest problem when it comes to communication tools like Slack: they can become really expensive quickly.
Slack offers various plans, each with its own features, but as your team grows, so does the total cost of ownership for a SaaS product.
For example, the Pro plan costs $7.25 per user per month, which might seem reasonable initially. However, when you have a large team, these costs can quickly escalate, leading to a significant monthly expense. At that price, you can find Slack alternatives that offer better features.
3. Slack bots need maintenance
Slack bots, much like any other chatbot, rely on the data they have been trained with. If a bot user asks for information that hasn’t been integrated into the bot’s system, it will likely fail to provide a satisfactory response.
This means that your Slack bot will require constant updates to stay relevant and useful.
You’ll need to keep track of the most common questions being asked and regularly feed your Slack bot new, relevant information. This ongoing maintenance can become a significant workload, especially as your Slack workspace grows.
4. Bots can eat up space
Slack has minimal storage capacity, and as these limits are reached, Slack automatically deletes older files and messages to make room for new ones.
This process can lead to the unintentional loss of important documents or communication history, especially if you’re using bots to automate tasks that generate a lot of data.
5. Slack bots aren’t creative
This is a no-brainer, but bots aren’t usually the smartest tool in the shed. They lack critical thinking and the ability to approach a situation from different perspectives.
Therefore, in a sticky situation where problem-solving is required, you cannot depend on your Slack bot to provide unique solutions.
Enhancing Team Communication with ClickUp and Slack
If you think Slack is an efficient communication tool, wait until you integrate it with ClickUp.
Together, Slack and ClickUp form the ultimate dream team in a collaborative environment.
Let’s explore how different teams can benefit from this integration while keeping Slack etiquettes in mind.
Enable ClickUp activity sync with Slack
Once you’ve integrated ClickUp with Slack, you can sync all your ClickUp activity with your Slack workspace for specific spaces, folders, and lists.
Your ClickUp activities will be automatically sent as messages to your Slack channel. You can select public or private channels where ClickUp can access these notifications.
To grant ClickUp access to a private channel, use the /invite @ClickUp command.
How does this help?
Imagine you’re part of a marketing team working on a high-stakes campaign with a tight deadline. This integration removes the need to update your team members on your progress manually.
Instead, ClickUp automatically sends updates to your Slack channel, giving everyone a 360-degree view of where the project currently stands.
Similarly, critical updates from the tech teams—like bug fixes, feature deployments, or server maintenance tasks—are immediately shared with the team in real time.
Tasks, comments, and docs, all on your Slack screen
When you link tasks, comments, or Docs in Slack, you’ll see a preview of each item, a process referred to as “unfurling.” This feature lets your team view details like task status, assignees, priorities, and more directly in Slack.
For instance, say a sales team has created a Slack channel to discuss a key account. When a task related to this account is shared on Slack, everyone can immediately see who’s assigned, what the priority is, and the current status.
Now, sales reps (or any other team) can respond quickly to client needs without digging through multiple apps.
Turn messages into tasks
You can create ClickUp tasks directly from Slack by using a /Slash command like /clickup new or adding a Slack message as a comment to an existing task.
This is particularly helpful when delegating tasks. Imagine the marketing head likes a creative idea shared in the Slack chat; they can instantly turn that message into a task in ClickUp without ever leaving Slack.
Also read: The DOs and DON’Ts of Using Slack at Work
ClickUp Does What Slack Can, and More
At the end of the day, you’re using Slack to communicate effectively with your team and across departments. But communication is more streamlined, effective, and intuitive on ClickUp. Let’s explore more.
Real-time conversations with chat view
ClickUp’s Chat View lets your team engage in real-time conversations without leaving the platform.
Whether you’re an IT team troubleshooting an urgent issue or a sales team discussing a new lead, the Chat View keeps all your communications centralized.
Marketing teams particularly struggle with scattered conversations across different apps. On ClickUp, you can brainstorm ideas, share creative briefs, and make quick decisions—all within the same workspace.
And when it’s time to turn those ideas into action, ClickUp lets you do it right from the chat. Assign tasks, set deadlines, and move projects forward without leaving the conversation.
Collaboration, brainstorming, and whiteboards
Need to brainstorm a strategy or plan a project? ClickUp’s Whiteboards offer a virtual space where teams can visually collaborate.
While Slack lets you brainstorm with your teammates through text-based discussions, ClickUp Whiteboards take your projects to the next level by allowing your team to draw, annotate, and turn ideas into actionable tasks on the board.
If you’re part of an engineering team that needs to map out workflows or a project manager handling a complex project timeline, this will become your new favorite tool.
Task management with Assigned Comments
One of ClickUp’s standout features is the ability to assign comments to specific team members using @mentions. If you’re discussing a critical update in Chat View, you can instantly tag a team member and turn that comment into a task.
For example, during a product development meeting, an engineering lead can assign a bug fix directly from the chat, ensuring nothing slips through the cracks.
Visual communication with ClickUp Clips
Are you a visual learner? If yes, ClickUp Clips is about to enhance your experience tenfold by allowing you to record and share screen recordings.
Imagine an IT team recording a step-by-step guide for troubleshooting a common issue—team members can access this clip anytime, making it easier for them to understand and implement changes.
Strategize actions with templates
At the end of the day, tools like ClickUp and Slack are communication enablers. For these tools to be useful and actually bring order to your internal communication, you need a strategy. And that’s where ClickUp’s Internal Communication Strategy and Action Plan Template can come in handy.
With this template, you can assess your existing communication strategy, chart out a new approach and its associated goals, set goals and objectives for your strategy, and create a coherent action plan.
You’re a Click Away from Better Communication with ClickUp
Slack is a great tool that becomes even better with ClickUp’s integration.
However, instead of juggling Slack messages and ClickUp texts at the same time, having one platform that can do it all makes more sense.
ClickUp’s real-time conversations, the convenience of turning one direct message into a detailed task, and the ability to visualize discussions—all make ClickUp a holistic project management app when compared to Slack’s project management capabilities.
Try it out yourself to see the difference. Create your free ClickUp account today and simplify your workflows!
Questions? Comments? Visit our Help Center for support.