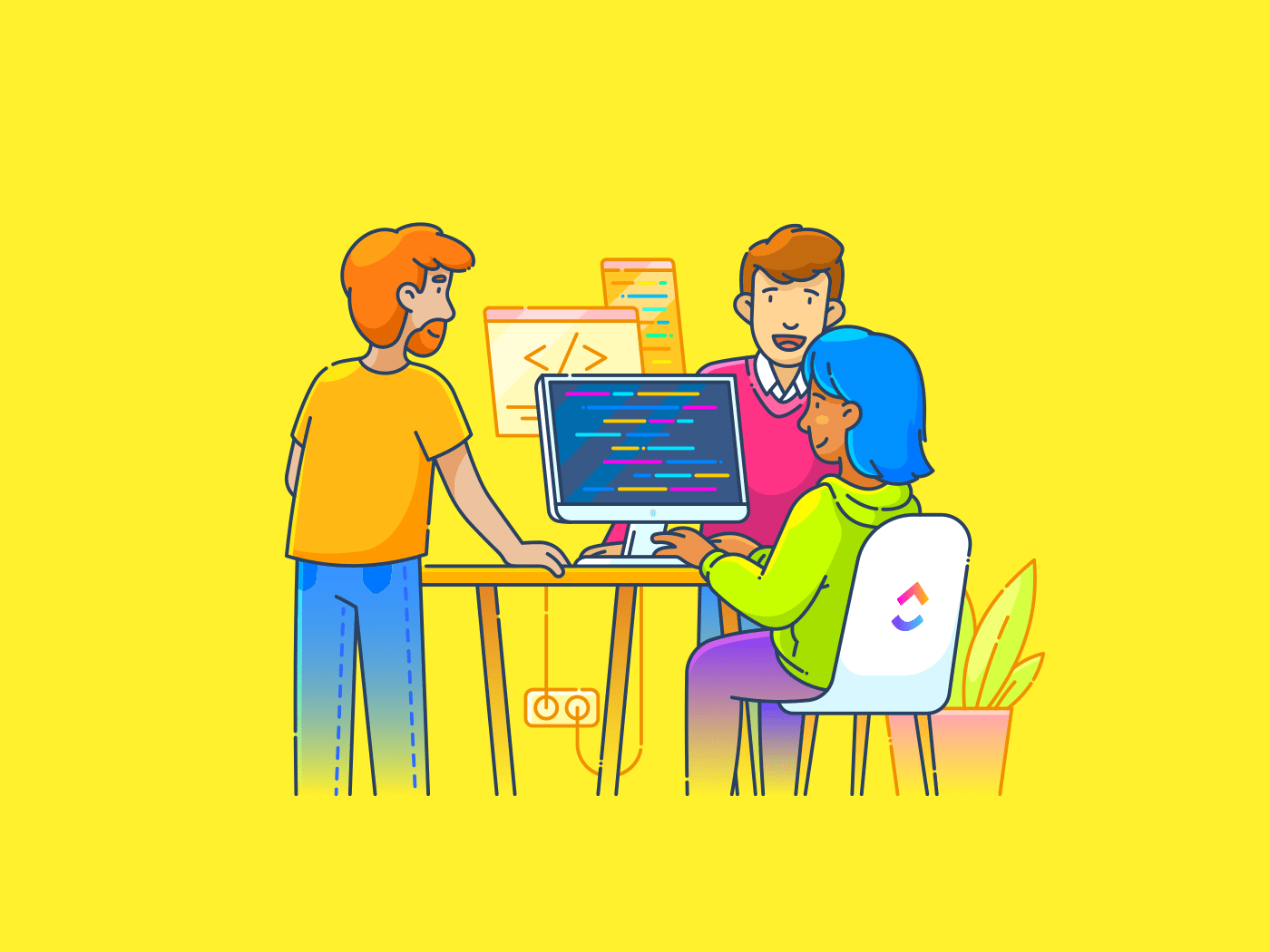
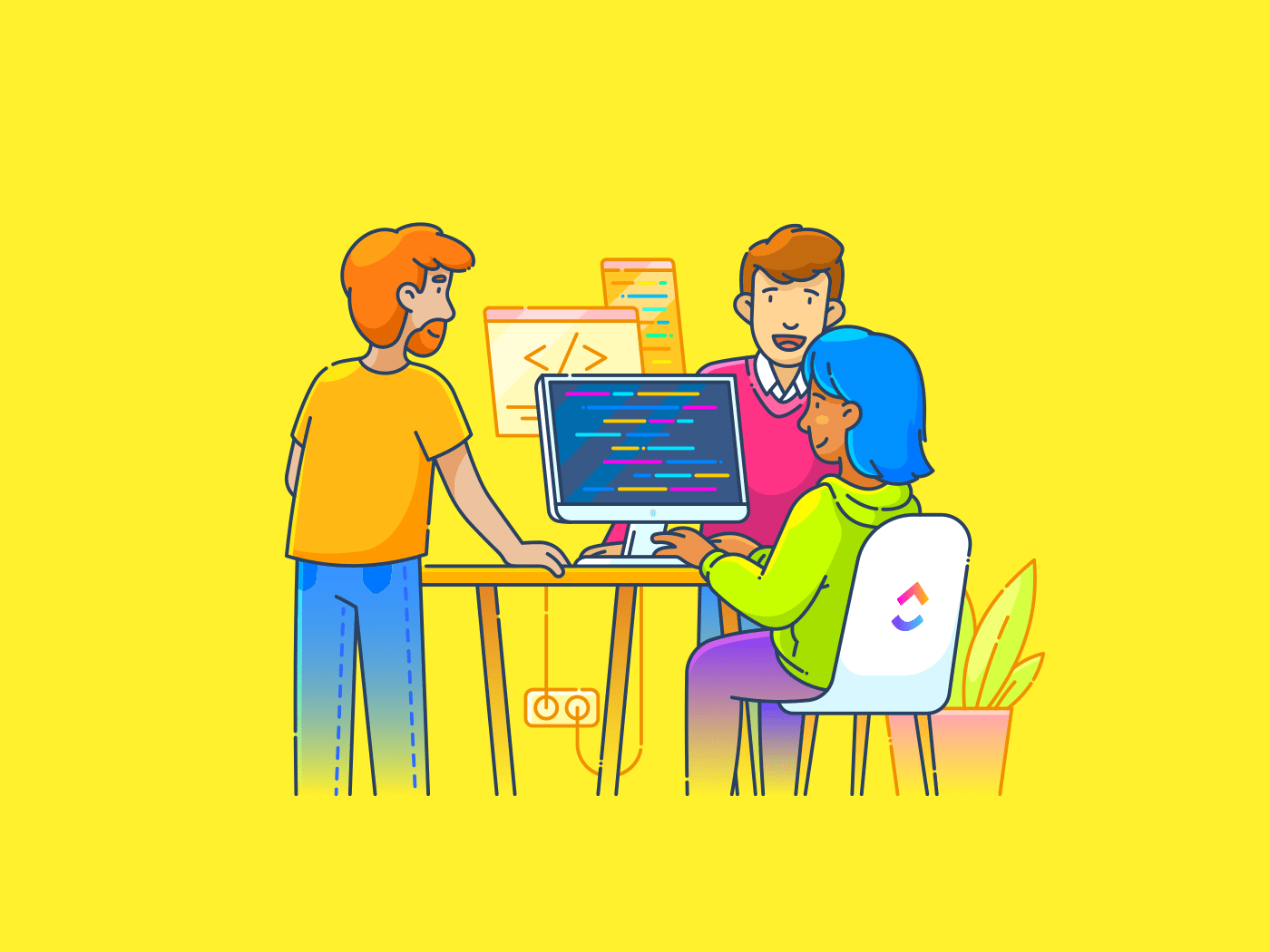
When you click a link, does it load instantly, or do you have to wait?
The difference between immediate and delayed responses is at the heart of how software handles tasks—and that’s where synchronous vs. asynchronous programming comes into play.
While synchronous programming works on one task at a time, asynchronous programming allows codes to multitask.
In modern software development, where time is everything, understanding when to use each method is crucial. This blog explores these programming approaches in detail and how you can use them. 🧑💻
Understanding Synchronous Programming
Synchronous programming, also known as blocking code, is a programming model in which users execute operations sequentially. While one task is in progress, others are paused, waiting their turn.
It follows a single-thread model, so tasks move in a strict order, one after the other. Once the current task is done, the next one can begin.
Synchronous tasks operate on a blocking architecture, making it ideal for reactive systems. The flow is linear, predictable, and easy to understand. A synchronous code structure can work well in some cases, but it might slow things down and cause delays when handling heavier tasks.
💡 Example: Synchronous coding is ideal for straightforward command-line tools such as file manipulation or basic arithmetic operations in a calculator application.
Let’s look at some scenarios where synchronous programming can help. 👇
- Data processing and analysis: It’s great for analysis that involves heavy computations and requires precise control over execution, such as scientific computation or statistical analysis
- Local database queries: Synchronous operations help create applications where database interactions are minimal and don’t need to handle multiple concurrent requests such as configuration requests, user profile retrieval, or inventory lookups
- Single-user desktop application: It works in applications for single-user environments, such as personal finance software and photo-editing apps
- Batch processing: It’s suitable for batch processing tasks where operations need a specific execution order, such as payroll processing, report generation, and data backup
- Event-driven programming: In certain real-time applications such as games or embedded systems, synchronous programming maintains timing and responsiveness
Understanding Asynchronous Programming
Asynchronous programming, also known as non-blocking code, is a programming model that allows multiple operations to run simultaneously without blocking the execution of other tasks.
It helps keep things moving smoothly, allowing processes to run in the background and reducing wait times.
Asynchronous programming is ideal for handling time-consuming operations, like simultaneous processing of multiple documents. JavaScript platforms, including browsers and Node.js, rely on this approach.
💡 Example: Instead of waiting, you can keep working in an application while a cursor loads. This makes the application more responsive, letting users flow through tasks without interruptions.
Here are some scenarios where asynchronous programming helps. 👇
- Web development: It’s used in web development to handle API requests without blocking the user interface
- File uploads and downloads: In applications that involve large file transfers, it prevents the app from becoming unresponsive
- Real-time applications: It’s ideal for chat services, allowing users to send and receive messages smoothly without freezing the interface, even when interacting with multiple users at once
- Web scraping: Asynchronous programming scrapes data from many websites at the same time to process multiple requests at once
- User interface responsiveness: It allows the UI to remain responsive when tasks such as generating responses take a long time
📌 Pro Tip: Leverage forms for software teams to support asynchronous programming and enable team members to submit feedback or requests without interrupting their workflow. You can set up real-time notifications for form submissions to facilitate synchronous discussions and ensure everyone stays updated.
Key Differences Between Synchronous and Asynchronous Programming
Understanding asynchronous vs. synchronous programming helps clarify how each approach handles tasks and time. It’s important to gauge how the two models differ:
- Build application programming interfaces (APIs)
- Create event-based architectures
- Decide how to handle long-running tasks
Let’s explore how they differ and where each one fits best. 📄
Criteria | Synchronous programming | Asynchronous programming |
Execution pattern | Executes one task after the other in a linear sequence | Executes many tasks concurrently without waiting for one to finish before starting another |
Blocking behavior | Uses a blocking approach | Uses a non-blocking approach |
Performance impact | Leads to inefficiencies in scenarios involving waiting as the system remains idle during task execution | Enhances efficiency and responsiveness, particularly for input-output bound operations |
User experience and responsiveness | Results in a less responsive user experience if a task takes too long, potentially freezing the software | Provides a more responsive user experience, allowing users to interact with the application |
Scalability | Lower scalability due to sequential processing; adding new tasks often requires significant changes to the codebase | Higher scalability as it handles multiple tasks simultaneously |
Complexity | Simpler structure; it’s easier to understand and implement for straightforward tasks | More complex; it requires an understanding of callbacks, promises, or async/await patterns, which can introduce bugs if not managed properly |
Overhead | Generally lower overhead | Higher overhead due to the need for managing asynchronous operations and potential context switching |
Debugging ease | Easier debugging due to predictable execution workflow; errors can be traced in a linear manner | More challenging debugging due to potential race conditions |
Use cases | Ideal for simple applications, scripts, or tasks | Best suited for I/O-bound tasks such as web requests, database queries, or applications needing real-time user interactions |
Resource utilization | Less efficient resource utilization | More efficient resource utilization |
Use Cases and Applications in the Real World
Asynchronous programming is like a multitasker, jumping between tasks and notifying the system once each is done. On the other hand, synchronous programming takes a one-track approach, completing each task in a strict, orderly sequence.
Choosing between the two depends on the type of application and the project’s needs. Each approach has unique features and advantages, making it useful in different situations.
Let’s look at some factors you must consider before you choose between the two. 💁
- Task nature: Asynchronous programming is better suited for applications that rely on input/output tasks, like web apps fetching data. On the other hand, for CPU-heavy tasks where order is important, synchronous programming may work best
- Complexity vs. performance: Asynchronous programming can boost performance and keep things responsive, though it adds complexity in handling errors and debugging. Synchronous code is simpler to manage but can be less efficient if not handled carefully
- Project size: Smaller projects may find synchronous programming simpler and easier to work with, while larger, scalable applications that need quick responses often benefit from an asynchronous approach
Here are some industry examples of synchronous programming:
- CPU-intensive operations: It’s effective for tasks that need significant CPU processing without extensive I/O wait times, such as mathematical computations
- Simple applications: Smaller projects such as arithmetic problems, simple games, and file manipulation
On the other hand, asynchronous programming is used for:
- Mobile development: It helps manage background operations and UI and makes API calls to fetch data
- Data streaming applications: It processes high volumes of data in real time, such as a stock ticker app, which continuously receives and displays stock prices live
📖 Also Read: 25 Software Development KPIs with Examples
Tools and Techniques for Implementing Asynchronous Programming
Synchronous programming works well for simple, linear tasks, but when apps need to stay responsive or handle multiple tasks at once, asynchronous programming steps in.
From here on, we’ll focus more on asynchronous techniques, which are essential for building efficient, scalable applications that can handle today’s demands.
To implement asynchronous programming effectively, developers have access to various tools and techniques that simplify managing tasks running simultaneously.
Learning these techniques helps one become a better programmer. You’ll learn how to streamline complex workflows, ensure efficient error handling, and provide greater control over task execution, making it easier to create scalable, user-friendly applications.
Let’s take a look at them together. 👀
JavaScript
Asynchronous JavaScript stands out for web development thanks to these key techniques:
- Callbacks: Functions that handle asynchronous events by executing once the task is completed. They’re simple but can lead to ‘callback hell’—a deeply nested structure that makes code harder to follow and maintain
- Promises: A significant improvement over callbacks, promises represent a future result of an asynchronous operation, whether resolved or rejected. They keep code more readable by preventing deeply nested callbacks and allow more structured handling of asynchronous workflows
- Async/await: Added in ES2017, this syntax helps software developers write asynchronous code that reads almost like synchronous code. Using async and await makes it easier to manage errors and simplifies complex asynchronous flows by eliminating promise chains and callback nesting
Each technique helps JavaScript effectively handle asynchronous tasks while keeping code maintainable and readable.
🔍 Did You Know? James Gosling, the creator of Java, was inspired by his love for coffee and chose the name while sipping the beverage! Originally, it was named ‘Oak’, but it was later changed to ‘Java’, evoking the rich coffee culture in Indonesia, where some of the best coffee beans are grown.
Python
Python enables asynchronous programming through its asyncio library, which includes:
- Coroutines: Defined using ‘async def,’ coroutines are functions that can pause execution with await, letting other tasks run simultaneously. This makes it easier to manage tasks that would otherwise block execution, like network requests
- Event loop: At the core of asyncio, the event loop handles the execution of coroutines and manages non-blocking I/O. It’s essential for building applications that handle multiple tasks at once, such as web servers capable of managing thousands of simultaneous requests with minimal delay
🔍 Did You Know? Guido van Rossum, one of the world’s most famous computer programmers, created Python as a hobby project during the Christmas holidays in 1989. He based the name on his favorite show, Monty Python’s Flying Circus, a comedy TV show on BBC!
C#
In C#, the task asynchronous pattern (TAP) strongly supports managing asynchronous programming with features like ‘async and await’.
This approach lets developers write code that reads sequentially but runs tasks concurrently, making it ideal for applications that perform a lot of I/O operations. It enhances responsiveness and performance.
Integrating asynchronous programming techniques with task management tools like ClickUp Software Team Management Software significantly enhances workflow efficiency. It minimizes bottlenecks, as team members can tackle tasks in parallel, making it easier to meet project deadlines and allocate resources effectively.
ClickUp also offers a variety of integrations, such as the PractiTest integration, to help software teams enhance quality and manage tasks asynchronously. These integrations let you automate updates, sync software development tools, and streamline handoffs, keeping work moving smoothly.
Challenges and Solutions
Asynchronous programming comes with unique software development challenges that can impact an application’s performance, reliability, and user experience.
Some common issues and their practical solutions have been highlighted below.
1. Challenge: Asynchronous code can create complex structures, making it harder to follow and maintain, especially for those unfamiliar with non-linear code flows.
✅ Solution: Use promises or ‘async/await’ syntax to structure asynchronous code in a way that’s easier to read, closely resembling synchronous code while keeping it non-blocking.
2. Challenge: Errors in asynchronous programming may arise unexpectedly, making them difficult to trace and debug.
✅ Solution: Set up centralized error-handling functions or middleware to catch and log errors consistently for easy debugging.
3. Challenge: Asynchronous tasks can trigger race conditions, where the order of task completion affects results in unintended ways.
✅ Solution: Use synchronization tools, like mutexes or semaphores, to control access to shared resources, ensuring that only one process interacts with the resource at a time.
With ClickUp, tackling asynchronous programming challenges gets a whole lot easier.
From task tracking to team collaboration, ClickUp keeps everything organized and everyone on the same page, so you can focus on building efficient, reliable apps without the usual headaches. It also supports collaborative software development and seamless communication between teams.
ClickUp task management
ClickUp’s task management tools simplify project organization to a whole new level. Let’s look at its features together.
ClickUp Tasks
ClickUp Tasks make it easy to organize and manage work, especially with the flexibility needed for asynchronous programming. You can break down complex projects into smaller tasks, assign them to team members, and set clear deadlines, allowing everyone to work independently without missing updates.
Each asynchronous task includes sections for descriptions, attachments, and comments, keeping everything team members need in one place.
ClickUp Task Priorities
In asynchronous programs, tasks operate independently, often requiring clear direction for prioritization—especially when processes are complex or involve multiple contributors working at different times.
ClickUp Task Priorities helps developers and teams manage these independent workflows with the capability to identify essential tasks and assign them appropriate priority levels.
For example, a team member is responsible for error-handling code in an asynchronous app. They log into ClickUp, filter by ‘Urgent’ tasks, and immediately know what needs tackling first, bypassing the need for direct communication or waiting for their teammates.
ClickUp Custom Task Statuses
ClickUp Custom Statuses help you design workflow stages that fit the unique rhythm of async work. They enhance transparency and keep your teammates aligned without needing constant real-time updates.
ClickUp lets you create detailed statuses, such as ‘Waiting in Review’, ‘Coding In Progress’, or ‘Follow-up Needed,’ to communicate each task’s current stage.
ClickUp Task Dependencies
ClickUp Task Dependencies let you organize asynchronous operations, especially in complex projects where specific tasks must be finished before others can begin.
Asynchronous programming thrives with task dependencies, allowing teams to set up workflows where, for example, data fetching completes before processing starts.
For instance, say you’re developing an application that fetches data from an API before displaying it in the user interface. You can set a dependency in ClickUp so that the UI rendering task depends on the data-fetching task. This way, the front-end task isn’t started until the user input data is available, preventing errors from attempting to display data before it’s fetched.
ClickUp Task Comments
ClickUp Task Comments offer a space for team members to leave detailed updates, context, and guidance, ensuring everyone is aligned even if they check in at different times.
With @mentions, you can direct a comment to specific team members, who will be notified when it’s their turn to jump in or review a task. This targeted approach keeps communication streamlined and focused, reducing back-and-forth emails and chat messages.
For instance, if a developer completes a critical step in an asynchronous process, they can @mention the next team member, who receives an instant notification to proceed with the task.
ClickUp Automations
ClickUp Automations helps keep asynchronous workflows smooth, taking on repetitive tasks and letting you focus on more strategic activities. It lets you create automation at various project levels, including in a specific Space, Folder, or List, so it aligns with your team’s workflow.
You can set triggers and actions to create workflows that automatically update task statuses, move tasks between stages, or send alerts when key changes happen.
This setup facilitates instant notifications and generates follow-up tasks as conditions change, reducing the need for manual updates.
📖 Also Read: ClickUp Changes the Game for Software Teams
Embrace the Right Development Flow With ClickUp
Choosing between synchronous and asynchronous programming can significantly impact your project’s performance.
Synchronous programming is straightforward and ideal for tasks that need to run in order, while asynchronous programming excels in handling multiple operations simultaneously.
To manage these workflows effectively, ClickUp offers robust task management features, helping teams collaborate seamlessly. You can streamline your projects (almost magically), whether you’re coding synchronously or asynchronously.
Sign up to ClickUp today!