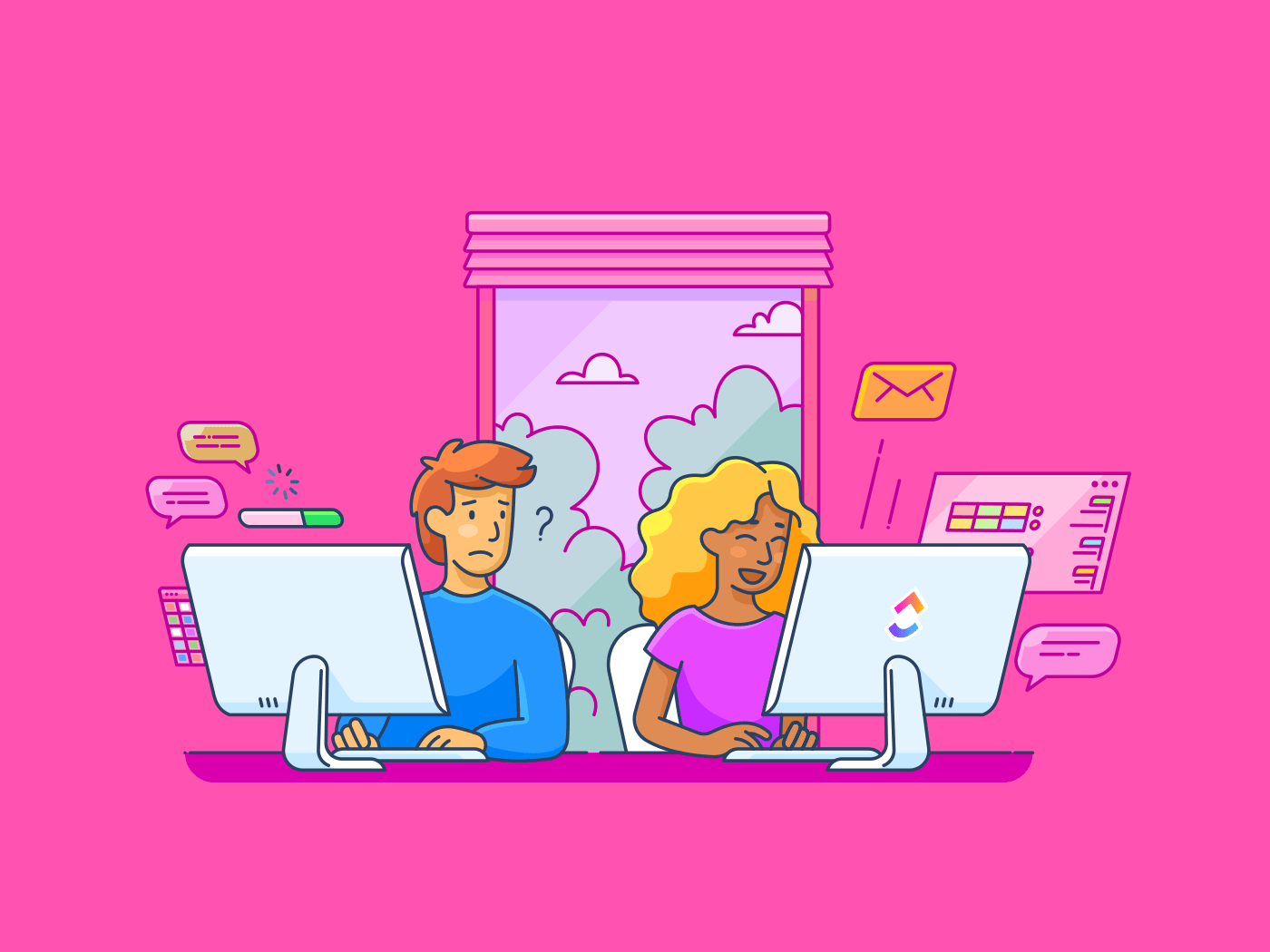
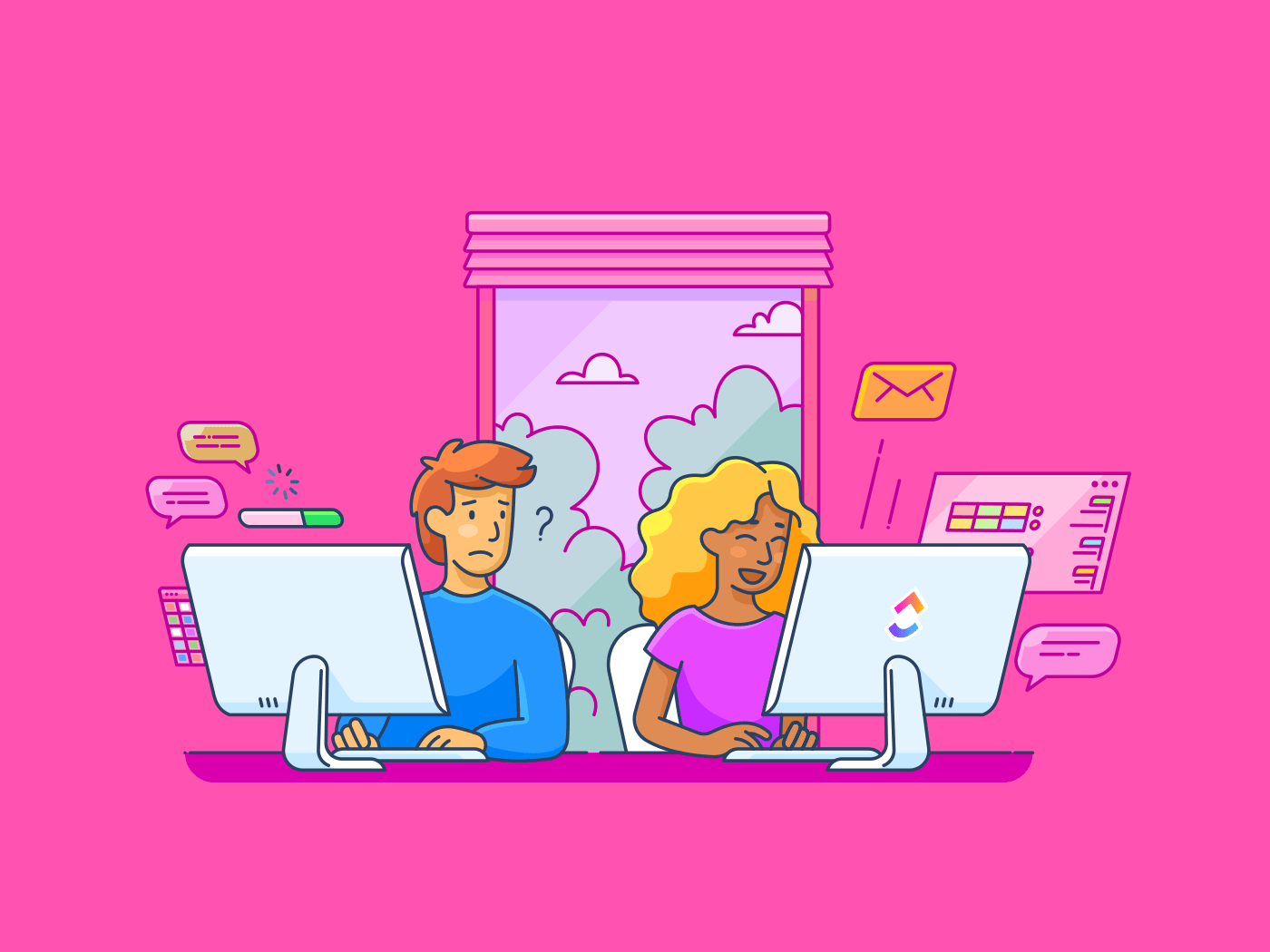
Hey there! Curious about how to spot all the hideouts of a certain substring within a larger string in Python? Well, you’re in luck! Let’s dive into this with a fun, easy-to-follow method that uses Python’s `find()` method. This little adventure in coding will not only solve your problem but also add a cool snippet to your toolkit!
Understanding the Task
Python strings come with a robust set of methods to manipulate and search through text. If you’re looking to find every single index where a particular substring starts within a string, you need a systematic way to do so, especially because Python’s built-in `find()` method only returns the first occurrence from a given starting point.
The Proposed Solution
To catch every occurrence, even those cunning overlapping ones, we can craft a custom function. This function will comb through the string from start to finish, catching every starting index where the substring pops up. Here’s how you can do it:
def find_all_indexes(input_string, substring):     start = 0     while True:         start = input_string.find(substring, start)         if start == -1:             return         yield start         start += len(substring) # Adjust this for overlapping substrings
How the Function Works
- Initialize the Start: We begin at the start of the string (index `0`).
- Search For The Substring: The `find()` method searches for the substring from the current `start` index.
- Return If Not Found: If `find()` doesn’t find the substring, it returns `-1`, which tells our function to stop the search.
- Yield the Index: If found, we `yield` (kind of like returning) the index.
- Move Forward: Increment the `start` index by the length of the substring to skip past this occurrence. To find overlapping substrings, you’d simply increment by 1 (`start += 1`).
Example in Action
Let’s see the function in a real scenario to better understand:
# Our test string and target substring input_string = "Pin and Spin at the inn." substring = "in" # Fetch and print all indices indexes = list(find_all_indexes(input_string, substring)) print(indexes)Â # What's the output here?
Output Explained:
When you run the above code, you should see:
[1, 11, 18, 21]
[1, 11, 18, 21]
These numbers represent the indices in `input_string` where the substring `”in”` begins. Note that there are some overlaps—pretty slick, right?
In Summary
This approach offers a versatile way to find substrings comprehensively within any string. Whether you’re handling data filtering, doing text analysis, or just having fun with strings, this function extends Python’s capability in a straightforward, efficient manner.
Feel free to play around with the increment in the loop to handle overlapping cases and see how the output changes. Happy coding, and remember, every line of code is a step towards mastering Python! 🚀
Unleashing ClickUp Brain to Solve Coding Challenges
At ClickUp, our goal is to make your work life simpler, more productive, and more joyful! We understand that coding problems can sometimes be tricky and time-consuming. That’s why we’ve integrated ClickUp Brain, our smart AI assistant, into the productivity suite to help you tackle these challenges effortlessly.
How ClickUp Brain Can Help With Coding Problems
- Explain Concepts: Struggling with understanding a particular function or method? Just ask ClickUp Brain! It can provide clear explanations and relevant coding examples to enhance your understanding.
- Debugging Assistance: Run into a snag with your code? Describe your problem to ClickUp Brain. It can suggest potential fixes and optimizations, guiding you through debugging processes step-by-step.
- Code Snippets: Need a quick function or two? Tell ClickUp Brain what you need. It will whip up code snippets directly in your workspace, saving you the time and hassle of searching through documentation.
- Learn Best Practices: ClickUp Brain stays updated with the latest coding standards and best practices. It can offer tips on how to write clean, efficient code that not only works but is also maintainable.
Using ClickUp Brain to Solve Substring Search:
Imagine you’re working on finding all occurrences of a substring within a string—the very problem we tackled earlier. Here’s how ClickUp Brain can turn into your coding companion:
- Activate ClickUp Brain: In your ClickUp workspace, go to the feature where ClickUp Brain is integrated, such as a task description or document.
- Describe Your Problem: Enter a description of your problem. For instance: “I need to find all starting indices of a substring in a Python string, including overlaps.”
- Review Suggestions: ClickUp Brain will process your request and provide a detailed, step-by-step solution or code snippets, just like the function we discussed. It may also offer alternative methods or additional tips.
- Implement and Refine: With the provided solution, implement it in your coding environment. If further refinements or explanations are needed, keep the conversation going with ClickUp Brain until you nail it!
ClickUp Brain is not just another tool; it’s your on-demand coding assistant designed to think, suggest, and assist actively. Whether you’re a seasoned developer or a newbie in the coding world, ClickUp Brain helps you focus more on creating and less on the hurdles. Say goodbye to coding frustrations and hello to streamlined, intelligent productivity with ClickUp Brain! 🧠✨
Happy coding, and remember, whenever you’re stuck, just a quick chat with ClickUp Brain can set you on the right path!